Documents
Create and manage forms that drivers fill out in the Samsara Driver App
Overview
Samsara Documents are custom forms that drivers can fill out in the Samsara Driver App. Fleet Administrators can manage documents and create custom document templates (called Document Types) in the Samsara Dashboard. Please see the Documents knowledge base article to understand how Documents work in the Samsara Dashboard and Driver App.
Here are the main tasks associated with Samsara's Documents API:
- Pull available Document Types that serve as a template for individual documents
- Create a document for a driver to fill out
- Configure a document submission webhook
- Pull submitted documents created or updated during a given time period
- Generate document PDF files
See below for details on Document Types and the Document Object.
Document Types
Document Types are templates that describe the structure of documents for drivers to fill out in the Driver App. For example, an organization might have an Accident document type, a Bill of Lading document type, a Citation document type, and many more.
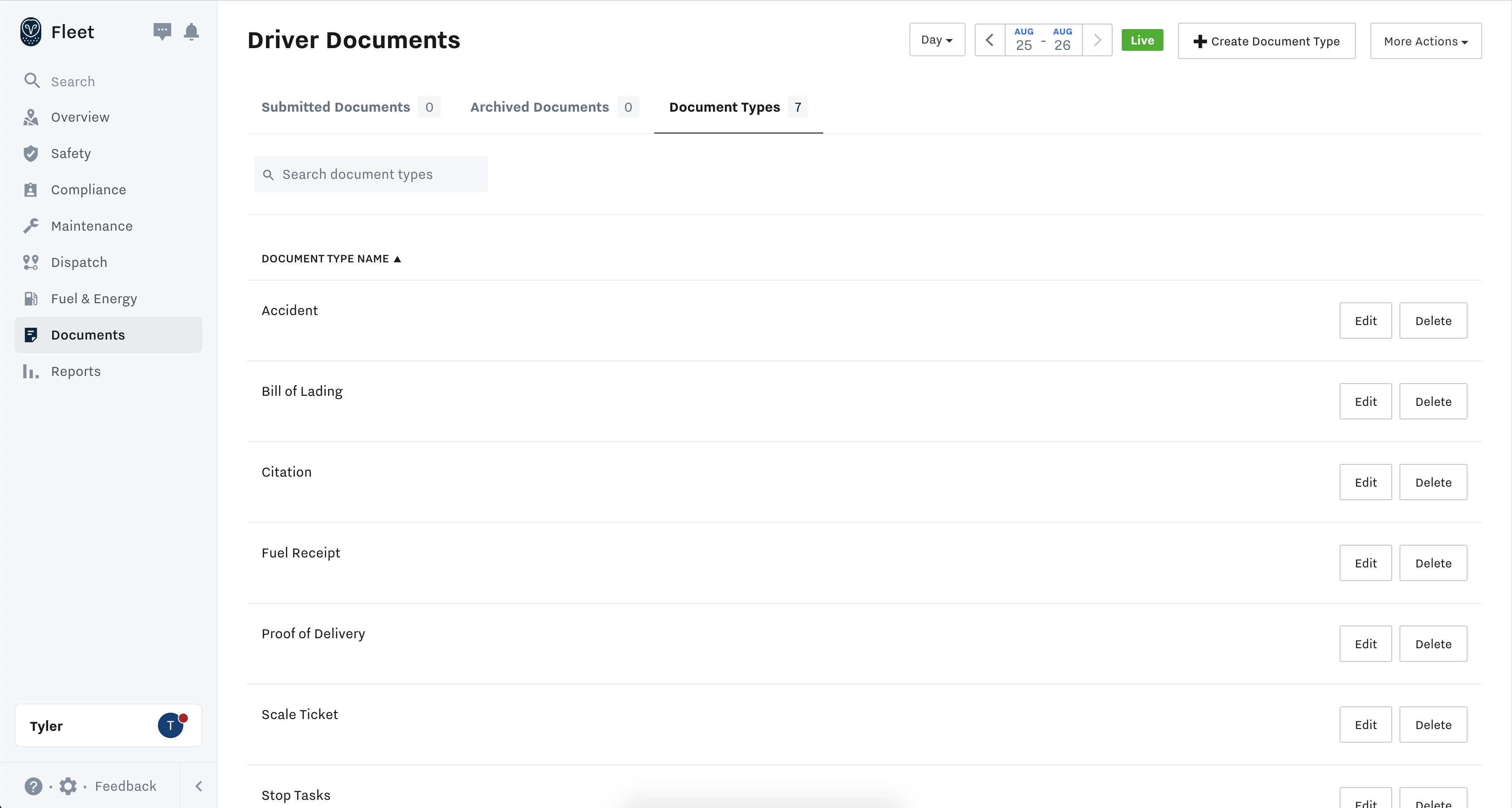
Document Types are managed in the Samsara Dashboard. Fleet managers can use out-of-the-box document types provided by Samsara, or they can define their own custom document types by defining the fields that make up that type of document.
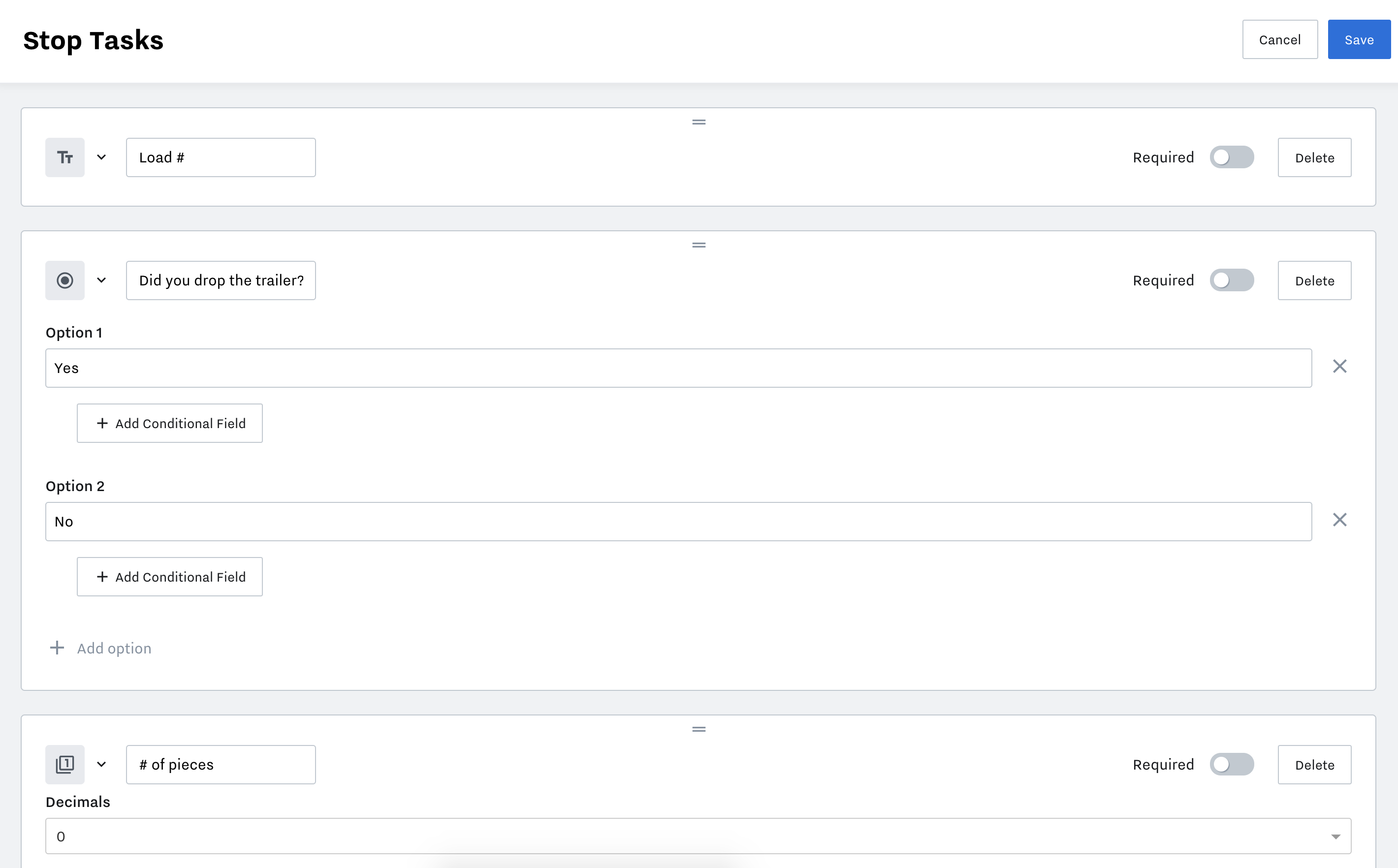
Retrieve document types via API
The Fetch document types API pulls all available documents types for your organization.
curl --request GET 'https://api.samsara.com/v1/fleet/drivers/document_types' \
--header 'Authorization: Bearer <<token>>'
{
"documentTypes": [
{
"orgId": 53729,
"uuid": "c3d04f1c-18f8-45fe-8c23-8f40f83851e0",
"name": "Stop Tasks",
"fieldTypes": [...]
},
...
]
}
Document Type Object:
Field | Description |
---|---|
orgId | The ID of the organization that created the document type. (Will always be the organization that the API token has access to). |
uuid | The universal identifier of the document type. |
name | Name of the document type. E.g., "Accident" , "Bill of Lading" , etc. |
fieldTypes | The fields that make up the document. E.g., "Load #" , "Did you drop the trailer?" , etc. This will include conditional fields. |
conditionalFieldSections | A description of which fields trigger conditional fields |
Field Types
The fieldTypes
array lists the fields that make up the document type. For example, you may have a string field named Load #
and a multiple choice field named Did you drop the trailer?
.
Fleet administrators can define the fields in the Samsara Dashboard.
Each field will have:
- A
label
which is the name of the field in the document. - A
valueType
which describes the type of this field. The available types that a field can have are:ValueType_Number
ValueType_String
ValueType_Photo
ValueType_MultipleChoice
ValueType_DateTime
ValueType_Signature
- Potential metadata depending on the value type:
numberValueTypeMetadata
- describes the number of decimals a numeric field can havemultipleChoiceValueTypeMetadata
- describes the options of a multiple choice fieldsignatureValueTypeMetadata
- provides legal text associated with the signature
Conditional Fields
When defining the document type, an admin can create conditional fields that appear only when the driver selects a certain multiple-choice option. In the example below, the "Photo of trailer" field only appears if the driver selects "Yes" to the multiple-choice question. If the driver selects "No", the signature field is presented instead.
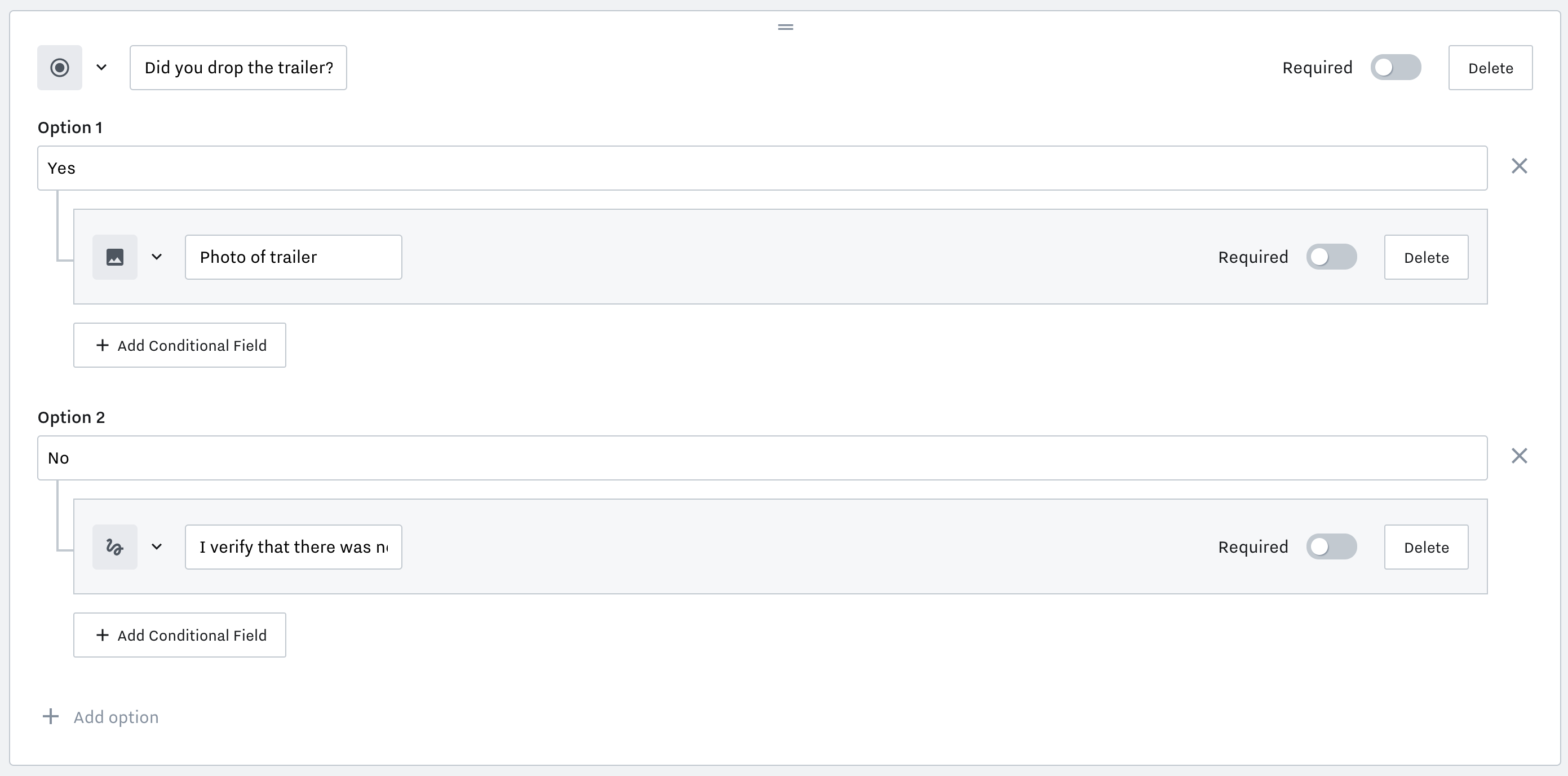
Conditional Fields
The definitions of the conditional fields are listed in the fieldTypes
array along with the all the other standard fields. The conditionalFieldSections
array lists the set of conditions that can trigger conditional fields.
"conditionalFieldSections": [
{
"triggeringFieldIndex": 1,
"triggeringFieldValue": "Yes",
"conditionalFieldFirstIndex": 2,
"conditionalFieldLastIndex": 2
},
{
"triggeringFieldIndex": 1,
"triggeringFieldValue": "No",
"conditionalFieldFirstIndex": 3,
"conditionalFieldLastIndex": 3
}
]
Each entry in the conditionalFieldSections
array describes a condition and a section of fields that are triggered by that condition.
In the example above, there are two conditions: selecting "Yes"
on the field with index 1
, and selecting "No"
on the field with index 1
.
The triggeringFieldIndex
indicates which field triggers the condition. The fieldTypes
array is 0-indexed, meaning index 0 refers to the first field in the array.
The triggeringFieldValue
indicates which multiple-choice value must be selected in order to trigger the condition.
Finally, the conditionalFieldFirstIndex
and conditionalFieldLastIndex
describe the section of the fieldsArray
that is activated by the condition.
Example
Let's take a Document Type that looks like this:
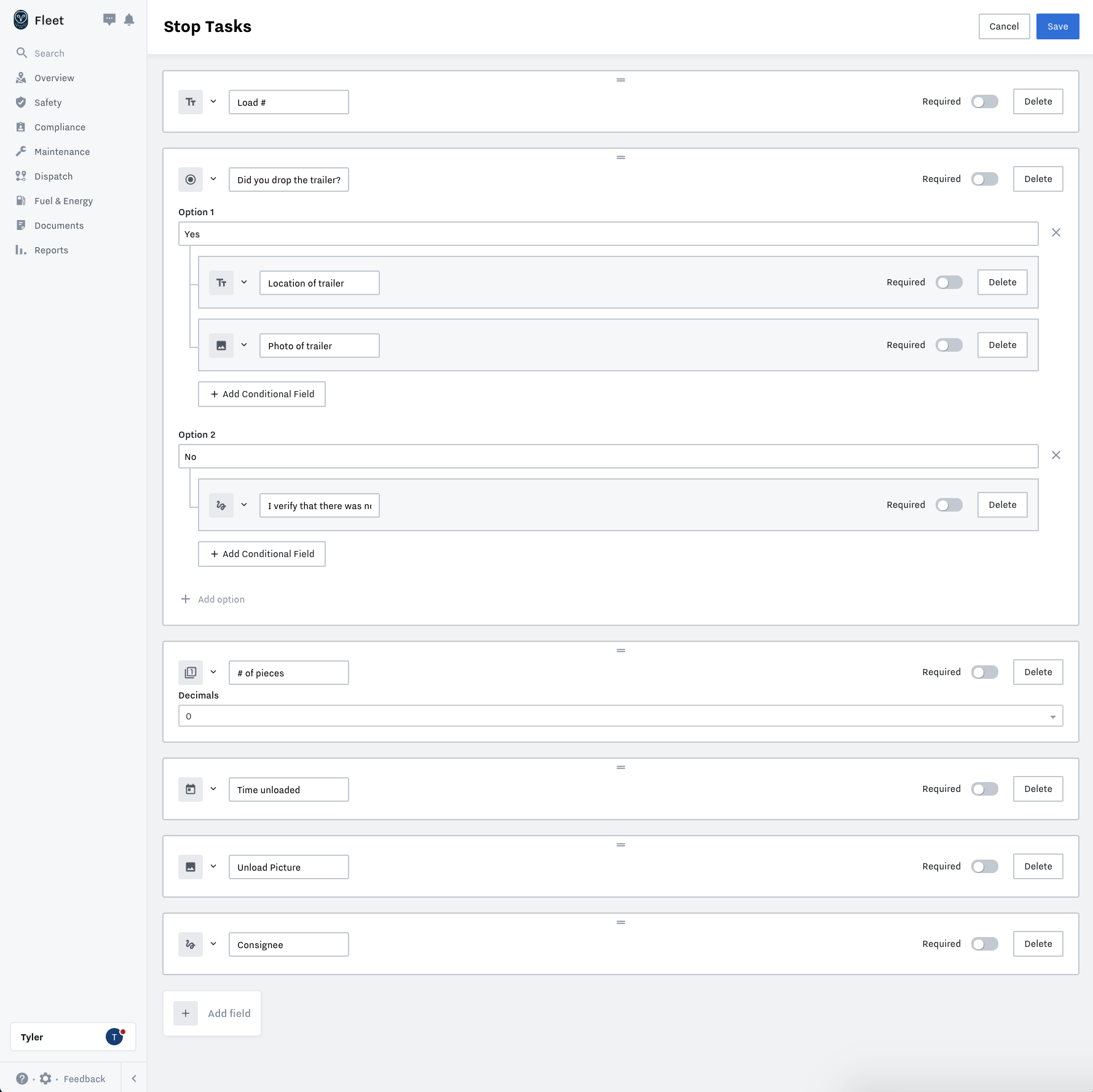
Custom Document Type in the Samsara Dashboard
A call to the document types API will retrieve this document type (and any others that might be in the organization).
curl --request GET 'https://api.samsara.com/v1/fleet/drivers/document_types' \
--header 'Authorization: Bearer <<token>>'
{
"documentTypes": [
{
"orgId": 53729,
"uuid": "c3d04f1c-18f8-45fe-8c23-8f40f83851e0",
"name": "Stop Tasks",
"fieldTypes": [
{
"label": "Load #",
"valueType": "ValueType_String"
},
{
"label": "Did you drop the trailer?",
"valueType": "ValueType_MultipleChoice",
"multipleChoiceValueTypeMetadata": {
"multipleChoiceOptionLabels": [
{
"label": "Yes"
},
{
"label": "No"
}
]
}
},
{
"label": "Location of trailer",
"valueType": "ValueType_String"
},
{
"label": "Photo of trailer",
"valueType": "ValueType_Photo"
},
{
"label": "I verify that there was no trailer to drop.",
"valueType": "ValueType_Signature",
"signatureValueTypeMetadata": {
"legalText": "I consent on behalf of myself and my employer to using electronic signatures in this transaction. I understand that I can request a copy of the signed documentation from the party requesting my signature."
}
},
{
"label": "# of pieces",
"valueType": "ValueType_Number",
"numberValueTypeMetadata": {
"numDecimalPlaces": 0
}
},
{
"label": "Time unloaded",
"valueType": "ValueType_DateTime"
},
{
"label": "Unload Picture",
"valueType": "ValueType_Photo"
},
{
"label": "Consignee",
"valueType": "ValueType_Signature",
"signatureValueTypeMetadata": {
"legalText": "I consent on behalf of myself and my employer to using electronic signatures in this transaction. I understand that I can request a copy of the signed documentation from the party requesting my signature."
}
}
],
"conditionalFieldSections": [
{
"triggeringFieldIndex": 1,
"triggeringFieldValue": "Yes",
"conditionalFieldFirstIndex": 2,
"conditionalFieldLastIndex": 3
},
{
"triggeringFieldIndex": 1,
"triggeringFieldValue": "No",
"conditionalFieldFirstIndex": 4,
"conditionalFieldLastIndex": 4
}
]
}
]
}
Documents
Documents are specific instances of a given document type. They are either created in the Driver App or via API. See Creating Documents for a guide on how to create documents via API.
Retrieve documents via API
Documents can be retrieved by one of the following API endpoints:
- Fetch all documents - Returns an array of document objects created or updated during a provided time range
- Fetches a document - Returns a document object by its ID
Document Object
Field | Description |
---|---|
orgId | The ID of the organization this document belongs to. (Will always be the organization the API token has access to.) |
driverId | The ID of the driver that submitted the document. |
id | The document's ID. |
driverCreatedAtMs | The Unix UTC timestamp in milliseconds of when the document was created in the Driver App. |
serverCreatedAtMs | The Unix UTC timestamp in milliseconds of when the document was uploaded to the Samsara server. |
serverUpdatedAtMs | The Unix UTC timestamp in milliseconds of when the document was last updated (and reflected on the Samsara server). |
dispatchJobId | The ID of a route stop this document is associated with. See Creating Stop Tasks and Capture Task Completion for more details. |
state | Valid values: Required , Submitted , Archived . Required documents are pre-populated documents for the Driver to fill out in the Driver App and have not yet been submitted. Submitted documents have been submitted by the driver in the Driver App or on behalf of the driver via API. Archived documents have been archived by the admin in the cloud dashboard. |
documentType | The name of the Document Type for this document. |
vehicleId | The ID of the vehicle that the driver had selected in the Driver App when the document was submitted. |
fields | The fields and values for the document. |
name | The name that the driver provided for this document. |
See the Fetches a document API docs for full details.
Fields
The fields
array of the document contains all the fields and their values. The structure of these fields is defined by the document's Document Type.
All fields have the following properties:
label
- This is the same as thelabel
defined by the given document type. For example,"Load #"
,"Did you drop the trailer?"
, etc.valueType
- This is the type of the field as defined by the given document type. Valid values include:ValueType_String
ValueType_MultipleChoice
ValueType_Number
ValueType_DateTime
ValueType_Photos
ValueType_Signature
- The actual value for the field. This name of this property depends on the field's value type: e.g.
stringValue
,multipleChoiceValue
, etc. See below for details. value
- This property is deprecated. It will match the property that is specific to the field's value type.
String Fields
Example:
{
"label": "Load #",
"valueType": "ValueType_String",
"stringValue": "123ABC",
"value": "123ABC"
}
Multiple Choice Fields
Example:
{
"label": "Did you drop the trailer?",
"valueType": "ValueType_MultipleChoice",
"multipleChoiceValue": [
{
"value": "Yes",
"selected": true
},
{
"value": "No",
"selected": false
}
],
"value": [
{
"value": "Yes",
"selected": true
},
{
"value": "No",
"selected": false
}
]
}
The multipleChoiceValue
property is an array representing which option of the multiple-choice field is selected.
The options are described by the multipleChoiceValueTypeMetadata
in the document's Document Type.
An option's value
is the name of that option, and selected
indicates whether that option is selected. (value
s map to label
s in the multipleChoiceValueTypeMetadata
).
Number Fields
Example:
{
"label": "# of pieces",
"valueType": "ValueType_Number",
"numberValue": 12,
"value": 12
}
The number of decimal places supported by the field is described by the numberValueTypeMetadata
in the document's Document Type.
Datetime Fields
Example:
{
"label": "Time unloaded",
"valueType": "ValueType_DateTime",
"dateTimeValue": {
"dateTimeMs": 1593643324000
},
"value": {
"dateTimeMs": 1593643324000
}
}
dateTimeMs
is measured in the number of milliseconds since the Unix epoch in UTC.
Photo Fields
Example:
{
"label": "Unload Picture",
"valueType": "ValueType_Photo",
"photoValue": [
{
"url": "https://samsara-driver-media-upload.s3.us-west-2.amazonaws.com/orgs/53729/drivers/1654973/69805b51-d89d-4e41-843a-c738dbb47de8/5D976520-6174-4C5C-8406-A0B9929DDF06.jpg?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=ASIA3LY3RNWSBUFUX77K%2F20200702%2Fus-west-2%2Fs3%2Faws4_request&X-Amz-Date=20200702T181200Z&X-Amz-Expires=86400&X-Amz-Security-Token=IQoJb3JpZ2luX2VjEMr%2F%2F%2F%2F%2F%2F%2F%2F%2F%2FwEaCXVzLXdlc3QtMiJIMEYCIQCUBRsF4EVksyiUDNTejLxZd%2Bz7QdVDpN%2F0Occ7FtvDDQIhAMh51EWmPifHVVnXb%2BSHiX3wfIpSVW00YHck%2FcOXYxv8KtoDCGMQAhoMNzgxMjA0OTQyMjQ0IgxxlEeEfyUYJevciU0qtwN1v0HccO12m6DtEiDe93pN1cQ58yFNSdb1XAP0MefwbArbQv1qHzp2MLxlWvFwMLdgBH4aHTy91QYouhtLk%2BJSxN0AWImvjtfXRjirV1i7sxnephH0ltg44kb2pGCa%2FP%2BHkU%2Bt1DKmrGmjJDlTH%2Br3qAu4tEWK6u4l6bFs8MnJYH%2FP4YZbXozJd4yTKSHuntyY6OGIqFq0LUiuCKDbCjVoce3kgrui61R4YoGfXAXJS4vyKsqmrUObAdb2M9XLn3YXDI%2BkUnnCH4ytyPFnKP6E3%2BdeBUvddkAVxu1BHhVH1tNHn%2Bl007NbvspM9ekAuG9ILCUO%2FLolPXBaKKcXPWG9XTBElVKJ3vl8oahnLktbluO1BmihtNQD93CgeEsZz99IEeDpEZGgHN%2FcOjQ6eHevTU7UrgNHKKa%2BIlUQkrQc1MR%2B3v%2BuqeQebAf2l7P1cydhNxR5qzma6HyN6cqnzbFpAIJ9K6QX34m9N6Y880wLss2n1YJS08PQv6F%2BDVJWl3cKmTa3g42%2BbQFVgKx%2F72kwYwdiKgAvROOW4h39jIks1XWWjuMso2sMysCyBF29D2txgpeQsDc5MPSy%2BPcFOu4BgIpcxNM9uNNub%2FnBG2Q3SLbs2yWyGnX12um%2BuCcFfLHUYaXxEdvXWlWZ9OKHa6%2B8tpc7W2kqhWrPRZSqFlu6wAAO1QG%2Btsm84cv9HL3j23duPLnnjnI3YbWVnbwaqPPyzhyqBYquOimzQ0WuYgN6LytSl2F0CCHk3oOTg5KMcXZGyNjv5ERZrBspxbDGk19DDRyhNDvqUWC8rzKVRjWEZqsIE3oJbxvm3nWAsvRVOWgdQQYVVXg3x7hwAcklIGaaebEQ5VLdQt1NdBYR5uP%2BVtm4xyTXruYo34TIJTxXuG8bJVstrRHobL5VdM00%2Fg%3D%3D&X-Amz-SignedHeaders=host&response-expires=2020-07-03T18%3A12%3A00Z&X-Amz-Signature=d96f037682f080d8de82c8c70cad53d49e5cfb5046c5a2406b6f4b88db06a538"
}
],
"value": [
{
"url": "https://samsara-driver-media-upload.s3.us-west-2.amazonaws.com/orgs/53729/drivers/1654973/69805b51-d89d-4e41-843a-c738dbb47de8/5D976520-6174-4C5C-8406-A0B9929DDF06.jpg?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=ASIA3LY3RNWSBUFUX77K%2F20200702%2Fus-west-2%2Fs3%2Faws4_request&X-Amz-Date=20200702T181200Z&X-Amz-Expires=86400&X-Amz-Security-Token=IQoJb3JpZ2luX2VjEMr%2F%2F%2F%2F%2F%2F%2F%2F%2F%2FwEaCXVzLXdlc3QtMiJIMEYCIQCUBRsF4EVksyiUDNTejLxZd%2Bz7QdVDpN%2F0Occ7FtvDDQIhAMh51EWmPifHVVnXb%2BSHiX3wfIpSVW00YHck%2FcOXYxv8KtoDCGMQAhoMNzgxMjA0OTQyMjQ0IgxxlEeEfyUYJevciU0qtwN1v0HccO12m6DtEiDe93pN1cQ58yFNSdb1XAP0MefwbArbQv1qHzp2MLxlWvFwMLdgBH4aHTy91QYouhtLk%2BJSxN0AWImvjtfXRjirV1i7sxnephH0ltg44kb2pGCa%2FP%2BHkU%2Bt1DKmrGmjJDlTH%2Br3qAu4tEWK6u4l6bFs8MnJYH%2FP4YZbXozJd4yTKSHuntyY6OGIqFq0LUiuCKDbCjVoce3kgrui61R4YoGfXAXJS4vyKsqmrUObAdb2M9XLn3YXDI%2BkUnnCH4ytyPFnKP6E3%2BdeBUvddkAVxu1BHhVH1tNHn%2Bl007NbvspM9ekAuG9ILCUO%2FLolPXBaKKcXPWG9XTBElVKJ3vl8oahnLktbluO1BmihtNQD93CgeEsZz99IEeDpEZGgHN%2FcOjQ6eHevTU7UrgNHKKa%2BIlUQkrQc1MR%2B3v%2BuqeQebAf2l7P1cydhNxR5qzma6HyN6cqnzbFpAIJ9K6QX34m9N6Y880wLss2n1YJS08PQv6F%2BDVJWl3cKmTa3g42%2BbQFVgKx%2F72kwYwdiKgAvROOW4h39jIks1XWWjuMso2sMysCyBF29D2txgpeQsDc5MPSy%2BPcFOu4BgIpcxNM9uNNub%2FnBG2Q3SLbs2yWyGnX12um%2BuCcFfLHUYaXxEdvXWlWZ9OKHa6%2B8tpc7W2kqhWrPRZSqFlu6wAAO1QG%2Btsm84cv9HL3j23duPLnnjnI3YbWVnbwaqPPyzhyqBYquOimzQ0WuYgN6LytSl2F0CCHk3oOTg5KMcXZGyNjv5ERZrBspxbDGk19DDRyhNDvqUWC8rzKVRjWEZqsIE3oJbxvm3nWAsvRVOWgdQQYVVXg3x7hwAcklIGaaebEQ5VLdQt1NdBYR5uP%2BVtm4xyTXruYo34TIJTxXuG8bJVstrRHobL5VdM00%2Fg%3D%3D&X-Amz-SignedHeaders=host&response-expires=2020-07-03T18%3A12%3A00Z&X-Amz-Signature=d96f037682f080d8de82c8c70cad53d49e5cfb5046c5a2406b6f4b88db06a538"
}
]
}
The photoValue
will be an array of photos, each containing a url
to the photo uploaded by the driver. The URLs expire after 24 hours, but you may retrieve the document again to get new URLs.
Signature Fields
Signatures cannot be submitted via the API. The value for a signature field must be left blank. Example:
{
"label": "Consignee",
"valueType": "ValueType_Signature",
"signatureValue": {
"name": "Tyler Freckmann",
"signedAtMs": 1593713506446,
"url": "https://samsara-driver-media-upload.s3.us-west-2.amazonaws.com/orgs/53729/drivers/1654973/84b927d5-c3c7-4cbf-803e-caac744b0bd2/driverMediaUpload-84b927d5-c3c7-4cbf-803e-caac744b0bd2?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=ASIA3LY3RNWSCW25HCS6%2F20200702%2Fus-west-2%2Fs3%2Faws4_request&X-Amz-Date=20200702T181200Z&X-Amz-Expires=86400&X-Amz-Security-Token=IQoJb3JpZ2luX2VjEMX%2F%2F%2F%2F%2F%2F%2F%2F%2F%2FwEaCXVzLXdlc3QtMiJGMEQCIAZ20xlWO6mJAh2RPLfQo97OFJyHsT%2FLQT5nZpUe%2FgBBAiBVAZPror%2BJy30jbsNNuVWiTvhyC1Z%2FOB9UB8HQPBCyySraAwheEAIaDDc4MTIwNDk0MjI0NCIMAq8tMRH1AVxv%2FLzzKrcDuNeUDSQpBnawbUP%2FI5xtjaejnJmbcVHu9vQ%2FhDcxF4%2Bu19VABvBhK6KPbO09WlbrbNO%2Fcqh1oWAZqmiJ5CtnQog3DUtaw%2BNmpFvuW5xva3blVSshXA%2B0slvdBJmNchR%2FyM4MmlzbWiEhbkp6puR03ZIdJl3yAWlB%2FBHlgw2FAl8AsbmP1FDY%2B%2Buhu9NxzPbnxK9400ow6VLLIZ5t98%2BrT2SLc3%2BcDtnGAvL1mlZ6DaOPe%2Fz%2B2Fth%2FCYD06Aj%2FewkiC3Y3oXoUXwzpZm4GNCbqBwBuKhD5L5IYGwGb%2B8zBb8nzCl%2BsIfemR4HeL72OMaM8Kjk32MPg0mbPRgMxZPnrPFcVOFf2fQLOyQsG4aZd3w%2FTjkGwZxiBUU2H8FrwFAYmYST13wPYKd2%2F7zYlkHGkD96PsxpDq33ChjvL9AZt%2Bd00jvCOlNz0i9b7Mdk9rCTn8idOMR5ArOciI02XID8OQsyFaurJgXUyRUWJZp4QOQ9AR16YF1EfdZNuq22DoNvGj%2FOOKEewkt42qYT5Yx2bOVFCCKUzpklZOmygXNU%2BT2QrV7dZKtDy6W1nGt3V9Z5Yq3R8nzblDCyt%2Ff3BTrwAZHFnrM7xu%2FJ1VB%2B0h%2FNj4nX6cQDTBAOXVwVdhfF1gXs6XB3VM177M6pFEwvF00ipn%2F4%2BzCPd2qgh36%2FzMVVAo7mr7BfuuD%2FGWWaZJKLqu1QLvpPlTAQXbHHh32RnWWs8e6o%2BdSzW%2BNWPEAbv91yt%2Fs8I5fv8XijhyPgmqfrhTXVQb9UHPsi1cS19HKhsNYcOWuZMlC2H%2FREPlJere65ZFrkijHQruV3cpdyDtkiBMJsjWeqxumLhYHLriG1iZ32LbAJozolygGtUg2%2BgPL7Uawl9pAQttcEKzqg4MVRzWMcHKBQAz%2BEUBQgZFehFUOG7A%3D%3D&X-Amz-SignedHeaders=host&response-expires=2020-07-03T18%3A12%3A00Z&X-Amz-Signature=01ef8a3af0e61f43bb2e5013a5a144c49b9ffcb07fc9148c87ecfba5fbdd8406"
},
"value": {
"name": "Tyler Freckmann",
"signedAtMs": 1593713506446,
"url": "https://samsara-driver-media-upload.s3.us-west-2.amazonaws.com/orgs/53729/drivers/1654973/84b927d5-c3c7-4cbf-803e-caac744b0bd2/driverMediaUpload-84b927d5-c3c7-4cbf-803e-caac744b0bd2?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Credential=ASIA3LY3RNWSCW25HCS6%2F20200702%2Fus-west-2%2Fs3%2Faws4_request&X-Amz-Date=20200702T181200Z&X-Amz-Expires=86400&X-Amz-Security-Token=IQoJb3JpZ2luX2VjEMX%2F%2F%2F%2F%2F%2F%2F%2F%2F%2FwEaCXVzLXdlc3QtMiJGMEQCIAZ20xlWO6mJAh2RPLfQo97OFJyHsT%2FLQT5nZpUe%2FgBBAiBVAZPror%2BJy30jbsNNuVWiTvhyC1Z%2FOB9UB8HQPBCyySraAwheEAIaDDc4MTIwNDk0MjI0NCIMAq8tMRH1AVxv%2FLzzKrcDuNeUDSQpBnawbUP%2FI5xtjaejnJmbcVHu9vQ%2FhDcxF4%2Bu19VABvBhK6KPbO09WlbrbNO%2Fcqh1oWAZqmiJ5CtnQog3DUtaw%2BNmpFvuW5xva3blVSshXA%2B0slvdBJmNchR%2FyM4MmlzbWiEhbkp6puR03ZIdJl3yAWlB%2FBHlgw2FAl8AsbmP1FDY%2B%2Buhu9NxzPbnxK9400ow6VLLIZ5t98%2BrT2SLc3%2BcDtnGAvL1mlZ6DaOPe%2Fz%2B2Fth%2FCYD06Aj%2FewkiC3Y3oXoUXwzpZm4GNCbqBwBuKhD5L5IYGwGb%2B8zBb8nzCl%2BsIfemR4HeL72OMaM8Kjk32MPg0mbPRgMxZPnrPFcVOFf2fQLOyQsG4aZd3w%2FTjkGwZxiBUU2H8FrwFAYmYST13wPYKd2%2F7zYlkHGkD96PsxpDq33ChjvL9AZt%2Bd00jvCOlNz0i9b7Mdk9rCTn8idOMR5ArOciI02XID8OQsyFaurJgXUyRUWJZp4QOQ9AR16YF1EfdZNuq22DoNvGj%2FOOKEewkt42qYT5Yx2bOVFCCKUzpklZOmygXNU%2BT2QrV7dZKtDy6W1nGt3V9Z5Yq3R8nzblDCyt%2Ff3BTrwAZHFnrM7xu%2FJ1VB%2B0h%2FNj4nX6cQDTBAOXVwVdhfF1gXs6XB3VM177M6pFEwvF00ipn%2F4%2BzCPd2qgh36%2FzMVVAo7mr7BfuuD%2FGWWaZJKLqu1QLvpPlTAQXbHHh32RnWWs8e6o%2BdSzW%2BNWPEAbv91yt%2Fs8I5fv8XijhyPgmqfrhTXVQb9UHPsi1cS19HKhsNYcOWuZMlC2H%2FREPlJere65ZFrkijHQruV3cpdyDtkiBMJsjWeqxumLhYHLriG1iZ32LbAJozolygGtUg2%2BgPL7Uawl9pAQttcEKzqg4MVRzWMcHKBQAz%2BEUBQgZFehFUOG7A%3D%3D&X-Amz-SignedHeaders=host&response-expires=2020-07-03T18%3A12%3A00Z&X-Amz-Signature=01ef8a3af0e61f43bb2e5013a5a144c49b9ffcb07fc9148c87ecfba5fbdd8406"
}
}
The signatureValue
will contain the name of the signature, the time of the signature measured in milliseconds since the Unix epoch in UTC, and a URL to PNG version of the signature. This URL expires after 24 hours, but you may retrieve the document again to get a new URL.
Updated over 2 years ago